To add a Facebook Like button to the TinyMCE editor, we need to use the setup callback function that enables us to add events to the editor instances before they get rendered.
Set 1: Add a Callback function in your TinyMCE init function
Add a callback function to your TinyMCE init function as follows. Make sure that all of your other configurations are taken care by separating each attribute values with comma (,)
And add this new button to tool bar as follows:
By Default TinyMCE will strip all invalid tags from editor, to add the Facebook tags as valid tags in TinyMCE add the following line..
The valid_elements option defines which elements will remain in the edited text when the editor saves
So, finally your TinyMCE init function looks like as follows
Step 2: Include the following JavaScript SDK of Facebook on your default Layout / Master page
That’s it; you’ll get a custom toolbar button in your TinyMCE editor!
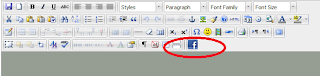
Set 1: Add a Callback function in your TinyMCE init function
Add a callback function to your TinyMCE init function as follows. Make sure that all of your other configurations are taken care by separating each attribute values with comma (,)
tinyMCE.init({
...
setup : function(ed) {
ed.addButton('facebook', {
title : 'Facebook Like',
image : '[Your path for image]/facebook.png',
onclick : function() {
ed.focus();
ed.selection.setContent('<fb:like send="false" width="450" show_faces="true"></fb:like>');
}
});
}
});
And add this new button to tool bar as follows:
theme_advanced_buttons4: “facebook"
By Default TinyMCE will strip all invalid tags from editor, to add the Facebook tags as valid tags in TinyMCE add the following line..
valid_elements: "fb:like[send|width|show_faces]"
The valid_elements option defines which elements will remain in the edited text when the editor saves
So, finally your TinyMCE init function looks like as follows
tinyMCE.init({
...
valid_elements: "fb:like[send|width|show_faces]",
setup : function(ed) {
ed.addButton('facebook', {
title : 'Facebook Like',
image : '[Your path for image]/facebook.png',
onclick : function() {
ed.focus();
ed.selection.setContent('<fb:like send="false" width="450" show_faces="true"></fb:like>');
}
});
},
theme_advanced_buttons4: “facebook"
});
Step 2: Include the following JavaScript SDK of Facebook on your default Layout / Master page
<div id="fb-root"></div>
<script>(function(d, s, id) {
var js, fjs = d.getElementsByTagName(s)[0];
if (d.getElementById(id)) return;
js = d.createElement(s); js.id = id;
js.src = "//connect.facebook.net/en_US/all.js#xfbml=1";
fjs.parentNode.insertBefore(js, fjs);
}(document, 'script', 'facebook-jssdk'));</script>
That’s it; you’ll get a custom toolbar button in your TinyMCE editor!
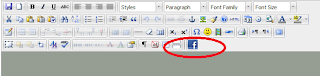